ECMAScript 2023, the 14th edition of the language, has some great changes that will make your programming life easier.
Thursday, November 30, 2023
Thursday, November 23, 2023
How to Create Web Component
As of 2023, the support for web components, alternatively referred to as custom elements, across browsers is exceptionally robust. Now is an opportune moment to embark on the journey of crafting your personalized elements for the web.
Web components, synonymous with custom elements, represent novel HTML elements that you can personally construct. These elements encapsulate a combination of markup, style, and interactive features.
Within the confines of this article, you'll delve into the fundamentals of web components and embark on the creation of a straightforward web component designed to display the current date.
Example
# A Complete Guide to Regular Expressions
Regular expressions, often referred to as regex or regexp, provide a powerful and flexible way to search, match, and manipulate text. They are widely used in programming, text processing, and data validation. This guide aims to provide a comprehensive overview of regular expressions, including syntax, common patterns, and practical examples.
## Table of Contents
- Introduction to Regular Expressions
- Basic Syntax and Matching
- Metacharacters
- Quantifiers
- Character Classes
- Anchors
- Grouping and Capturing
- Alternation
- Escape Characters
- Examples and Use Cases
### 1. Introduction to Regular Expressions
Regular expressions are patterns that describe sets of strings. They are used for pattern matching within strings. A regular expression is composed of ordinary characters and special characters called metacharacters.
### 2. Basic Syntax and Matching
- `.` (dot): Matches any single character except a newline.
- `^`: Anchors the regex at the start of a line.
- `$`: Anchors the regex at the end of a line.
Example:
^Hello$
This regex matches the string "Hello" only if it appears at the beginning of a line.
### 3. Metacharacters
- `*`: Matches zero or more occurrences of the preceding character or group.
- `+`: Matches one or more occurrences of the preceding character or group.
- `?`: Matches zero or one occurrence of the preceding character or group.
Example:
\d+
This regex matches one or more digits.
### 4. Quantifiers
- `{n}`: Matches exactly n occurrences of the preceding character or group.
- `{n,}`: Matches n or more occurrences of the preceding character or group.
- `{n,m}`: Matches between n and m occurrences of the preceding character or group.
Example:
\w{3,6}
This regex matches word characters (alphanumeric + underscore) with a length between 3 and 6.
### 5. Character Classes
- `\d`: Matches any digit (0-9).
- `\w`: Matches any word character (alphanumeric + underscore).
- `\s`: Matches any whitespace character (space, tab, newline).
Example:
[A-Za-z]\d{2}
This regex matches an uppercase or lowercase letter followed by two digits.
### 6. Anchors
- `\b`: Word boundary.
- `\B`: Non-word boundary.
- `^` (caret) and `$` (dollar): Match the start and end of a line, respectively.
Example:
\bword\b
This regex matches the word "word" as a whole word.
### 7. Grouping and Capturing
- `()`: Groups characters together.
- `(?:)`: Non-capturing group.
Example:
(\d{3})-(\d{2})
This regex captures a three-digit group, a hyphen, and a two-digit group.
### 8. Alternation
- `|`: Acts like a logical OR.
Example:
cat|dog
This regex matches either "cat" or "dog".
### 9. Escape Characters
- `\`: Escapes a metacharacter, treating it as a literal character.
Example:
\d\.\d
This regex matches a digit followed by a literal dot and another digit.
### 10. Examples and Use Cases
- Email Validation:
^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$
- URL Matching:
^(https?|ftp)://[^\s/$.?#].[^\s]*$
- Phone Number Matching:
^\+?[1-9]\d{1,14}$
- Extracting Date from Text:
(\d{4})-(\d{2})-(\d{2})
- HTML Tag Matching:
<([a-zA-Z][a-zA-Z0-9]*)\b[^>]*>(.*?)</\1>
Remember that while regular expressions are powerful, they can be complex. It's essential to test and validate them thoroughly.
This guide provides a foundation for understanding regular expressions, but there is much more to explore. Practice and experimentation are key to mastering this powerful tool.
Monday, November 20, 2023
CSOM vs JSOM vs SSOM vs REST
CSOM (Client-Side Object Model), JSOM (JavaScript Object Model), SSOM (Server-Side Object Model), and REST (Representational State Transfer) are different approaches or models used in SharePoint development for interacting with SharePoint data and functionality. Each has its own characteristics, advantages, and use cases. Here's an overview of each:
1. CSOM (Client-Side Object Model):
- Language Support: Primarily used with .NET languages such as C#.
- Execution Environment: Runs on the client side, usually within a .NET application.
- Usage: Ideal for scenarios where the code needs to be executed on the client side (e.g., in a Windows Forms application, WPF application, or a console application).
2. JSOM (JavaScript Object Model):
- Language Support: Primarily used with JavaScript.
- Execution Environment: Runs on the client side, typically in a browser.
- Usage: Well-suited for scenarios where client-side code (e.g., in a web page) needs to interact with SharePoint data and services.
3. SSOM (Server-Side Object Model):
- Language Support: Primarily used with .NET languages such as C#.
- Execution Environment: Runs on the server side, within the context of a SharePoint server.
- Usage: Typically used in scenarios where server-side code is necessary (e.g., in custom SharePoint server-side solutions, timer jobs, and event receivers).
4. REST (Representational State Transfer):
- Language Support: This can be used with a variety of languages, as it relies on HTTP and standard web technologies.
- Execution Environment: Runs on the client side or server side, depending on the implementation.
- Usage: Suitable for scenarios where a lightweight and language-agnostic approach is required. Can be used in web applications, mobile apps, or any scenario where HTTP requests can be made.
Key Points:
- CSOM and JSOM: Both are client-side models but are tailored to different development environments and languages.
- SSOM: Runs on the server side and is more suitable for server-side SharePoint solutions.
- REST: Offers a flexible and lightweight approach, making it suitable for a variety of scenarios and programming languages.
When choosing between these models, developers often consider factors such as the type of application, the development environment, and the specific requirements of the SharePoint solution being developed. Additionally, with the evolution of SharePoint and the emphasis on modern development practices, REST and client-side models like CSOM and JSOM are often preferred for their flexibility and compatibility with various platforms.
Wednesday, November 15, 2023
Get HTML table column values in array using map ()
Developers often find themselves needing to validate HTML table columns values, occasionally getting stuck in loops. Here's a straightforward solution: use the map() function to easily retrieve the values in an array.
SrNo | Name | City |
---|---|---|
1 | Rakesh | Vadodara |
2 | Anil | Ahmedabad |
3 | Sunil | Anand |
4 | Bharat | Udaipur |
Wednesday, November 8, 2023
Some Useful APIs
Sharing some valuable APIs that have been instrumental in my projects on this blog for your reference.
(1) LocalStorage API
It lets you store data against the website address in the form of key/value pairs. Local storage is limited to 5 MB.
(2) Clipboard API
This API provides access to the operating system's clipboard. You can use it to build a copy button to copy/paste content.
(3) Geolocation API
This API provides the user's geographical latitudes and longitudes and the accuracy to which it is correct.
(4) History API
This API lets you go back and forth between web pages that are in your session history.
(5) Fetch API
It is a browser API that lets you call REST APIs or GraphQL APIs.
Note: Content published here is sourced from social platforms, and full credit goes to the original author.Wednesday, October 18, 2023
Visual Studio Code CheatSheet
Visual Studio Code CheatSheet
This cheat sheet was a lifesaver for me; I hope it extends a helping hand to others too.
BASICS | |
Ctrl+Shift+N | New window/instance |
Ctrl+Shift+W | Close window/instance |
Ctrl+Shift+P or F1 | Show Command Palette |
Ctrl+P | Quick Open, Go to File |
Ctrl+, | User Settings |
Ctrl+K Ctrl+S | Keyboard Shortcuts |
Ctrl+C | Copy line (empty selection) |
Ctrl+X | Cut line (empty selection) |
Alt+ ↑ / ↓ | Move line up/down |
Shift+Alt + ↓ / ↑ | Copy line up/down |
Ctrl+Shift+K | Delete line |
Ctrl+Shift+Enter | Insert line above |
Ctrl+Home | Go to beginning of file |
Ctrl+End | Go to end of file |
Home / End | Go to beginning/end of line |
Ctrl+↑ / ↓ | Scroll line up/down |
Ctrl+Shift+\ | Jump to matching bracket |
Ctrl+Enter | Insert line below Basic editing |
Ctrl+] / [ | Indent/outdent line |
Ctrl+Shift+[ | Fold (collapse) region |
Ctrl+Shift+] | Unfold (uncollapse) region |
Alt+PgUp / PgDn | Scroll page up/down Basic editing |
Ctrl+/ | Toggle line comment |
Alt+Z | Toggle word wrap |
Ctrl+K Ctrl+U | Remove line comment Navigation |
Ctrl+T | Show all Symbols |
Ctrl+P | Go to File... |
Ctrl+K Ctrl+[ | Fold (collapse) all subregions |
Ctrl+K Ctrl+0 | Fold (collapse) all regions |
Ctrl+K Ctrl+J | Unfold (uncollapse) all regions |
Ctrl+K Ctrl+] | Unfold (uncollapse) all subregions |
Ctrl+K Ctrl+C | Add line comment |
Ctrl+Shift+O | Go to Symbol |
Ctrl+G | Go to Line Navigation |
Ctrl+Shift+M | Show Problems panel |
Shift+F8 | Go to previous error or warning |
Ctrl+Shift+Tab | Navigate editor group history |
F8 | Go to next error or warning |
EDITOR | |
Ctrl+K Ctrl+ ←/→ | Focus into previous/next editor group |
Ctrl+K ← / → | Move active editor group |
Ctrl+F4, Ctrl+W | Close editor |
Ctrl+\ | Split editor |
Ctrl+ 1 / 2 / 3 | Focus into 1st, 2nd or 3rd editor group |
Ctrl+K F | Close folder |
Ctrl+Shift+PgUp / PgDn | Move editor left/right |
Ctrl+M | Toggle Tab moves |
Shift+Alt+A | Toggle block comment |
Ctrl+N | New File |
Ctrl+S | Save |
Ctrl+Shift+S | Save As |
Ctrl+O | Open File |
Ctrl+K S | Save All |
Ctrl+K Ctrl+W | Close All |
Ctrl+Shift+T | Reopen closed editor |
Ctrl+F4 | Close |
Ctrl+K | Enter Keep preview mode editor open |
Ctrl+Shift+Tab | Open previous |
Ctrl+K P | Copy path of active file |
Ctrl+Tab | Open next |
Ctrl+K R | Reveal active file in Explorer |
Ctrl+K O | Show active file in new window/instance |
F11 | Toggle full screen |
Ctrl+Shift+E | Show Explorer / Toggle focus |
Ctrl+Shift+G | Show Source Control |
Ctrl+Shift+D | Show Debug |
Ctrl+Shift+F | Show Search Display |
Ctrl+Shift+X | Show Extensions |
Ctrl+Shift+J | Toggle Search details |
Ctrl+Shift+U | Show Output panel |
Ctrl+Shift+H | Replace in files |
Ctrl+Shift+V | Open Markdown preview |
Shift+Alt+0 | Toggle editor layout (horizontal/vertical) |
Ctrl+K Z | Zen Mode (Esc, Esc to exit) |
Ctrl+K V | Open Markdown preview to the side |
F5 | Start/Continue Debug |
F9 | Toggle breakpoint |
F10 | Step over |
Shift+F5 | Stop |
F11 / Shift+F11 | Step into/out |
Ctrl+K Ctrl+I | Show hover Integrated terminal |
Ctrl+` | Show integrated terminal |
Ctrl+C | Copy selection |
Ctrl+V | Paste into active terminal |
Ctrl+Shift+` | Create new terminal Integrated terminal |
Ctrl+↑ / ↓ | Scroll up/down |
Ctrl+Home / | End Scroll to top/bottom |
Shift+PgUp / PgDn | Scroll page up/down |
SEARCH AND REPLACE | |
Ctrl+F | Find |
F3 / Shift+F3 | Find next/previous |
Alt+Enter | Select all occurences of Find match |
Ctrl+H | Replace Search and replace |
Ctrl+D | Add selection to next find match |
Alt+C / R / W | Toggle case-sensitive / regex / whole word |
Ctrl+K Ctrl+D | Move last selection to next find match |
MULTI CURSOR SELECTION | |
Alt+Click | Insert cursor |
Ctrl+U | Undo last cursor operation |
Shift+Alt+I | Insert cursor at end of each line selected |
Ctrl+Alt+ ↑ / ↓ | Insert cursor above / below |
Ctrl+L | Select current line |
Ctrl+F2 | Select all occurrences of current word |
Shift+Alt+ → | Expand selection |
Ctrl+Shift+L | Select all occurrences of current selection |
Shift+Alt+ ← | Shrink selection |
Ctrl+Shift+Alt + (arrow key) | Column (box) selection |
Ctrl+Shift+Alt + PgUp/PgDn | Column (box) selection page up/down |
Shift+Alt + (drag mouse) | Column (box) selection |
LANGUAGE EDITING | |
F2 | Rename |
F12 | Go to Definition |
Ctrl+K F12 | Open Definition to the side |
Ctrl+. | Quick Fix |
Alt+F12 | Peek Definition |
Ctrl+Space, Ctrl+I | Trigger suggestion |
Shift+Alt+F | Format document |
Ctrl+K Ctrl+F | Format selection |
Ctrl+Shift+Space | Trigger parameter hints |
Shift+F12 | Show References |
Ctrl+K Ctrl+X | Trim trailing whitespace |
Ctrl+K M | Change file language |
Monday, October 9, 2023
Extract data from HTML table in an array using jQuery.
Individuals encounter challenges working with data in HTML when attempting to integrate it into their code. The following presents a straightforward method for extracting data from an HTML table and storing it in an array.
Monday, September 18, 2023
SharePoint Useful URLs for administrators and super users
This post serves as a valuable reference hub for all the handy shortcut URLs in SharePoint, streamlining tasks for administrators and super users.
While others have shared these URLs in various blog posts, I aim to safeguard them within my own blog, ensuring their accessibility for future generations!
Frequently used
Title | URL |
---|---|
Remove nav bar, header & command bar | ?env=WebView (after .aspx) |
Remove nav bar & header | ?env=Embedded |
Open list/ library in Microsoft Lists | ?env=WebViewList |
Site contents |
/_layouts/15/viewlsts.aspx /_layouts/15/viewlsts.aspx?view=14 (old style view) |
Manage content & structure | /_layouts/sitemanager.aspx |
Audit log reports | /_layouts/Reporting.aspx?Category=Auditing |
Shared with us | /Shared%20Documents/Forms/AllItems.aspx?view=3 |
Users and permissions
Title | URL |
---|---|
People | /_layouts/people.aspx |
Groups | /_layouts/groups.aspx |
Site Collection Admins | /_layouts/mngsiteadmin.aspx |
Advanced Permissions | /_layouts/user.aspx |
Galleries
Title | URL |
---|---|
Site Columns | /_layouts/mngfield.aspx |
Site content types | /_layouts/mngctype.aspx |
Web parts | /_catalogs/wp/Forms/AllItems.aspx |
List templates | /_catalogs/lt/Forms/AllItems.aspx |
Master Pages | /_layouts/ChangeSiteMasterPage.aspx |
Themes | /_catalogs/theme/Forms/AllItems.aspx |
Solutions | /_catalogs/solutions/Forms/AllItems.aspx |
Site administration
Title | URL |
---|---|
Regional settings | /_layouts/regionalsetng.aspx |
Content and structure | /_Layouts/sitemanager.aspx?Source={WebUrl}_layouts/settings.aspx |
Content and structure logs | /_Layouts/SiteManager.aspx?lro=all |
Site libraries and lists | /_layouts/mcontent.aspx |
User alerts | /_layouts/sitesubs.aspx |
RSS | /_layouts/siterss.aspx |
Search and offline availability | /_layouts/srchvis.aspx |
Sites and workspaces | /_layouts/mngsubwebs.aspx |
Workflows | /_layouts/wrkmng.aspx |
Workflow settings | /_layouts/wrksetng.aspx |
Related Links scope settings | /_layouts/RelLinksScopeSettings.aspx |
Content Organizer Settings | /_layouts/DocumentRouterSettings.aspx?Source=settings.aspx |
Content Organizer Rules | /RoutingRules/Group%20by%20Content%20Type.aspx |
Site output cache | /_Layouts/areacachesettings.aspx |
Term store management | /_Layouts/termstoremanager.aspx |
Taxonomy Hidden List | /Lists/TaxonomyHiddenList |
Searchable columns | /_Layouts/NoCrawlSettings.aspx |
Look and feel
Title | URL |
---|---|
Welcome page | /_Layouts/AreaWelcomePage.aspx |
Title, description and icon | /_layouts/prjsetng.aspx |
Master page | /_Layouts/ChangeSiteMasterPage.aspx |
Page layouts and site templates | /_Layouts/ChangeSiteMasterPage.aspx |
Tree view | /_layouts/navoptions.aspx |
Site theme | /_layouts/themeweb.aspx |
Navigation | /_layouts/AreaNavigationSettings.aspx |
Site actions
Title | URL |
---|---|
Manage site features | /_layouts/ManageFeatures.aspx |
Reset to site definition | /_layouts/reghost.aspx |
Delete this site | /_layouts/deleteweb.aspx |
Site web analytics reports | /_layouts/WebAnalytics/Report.aspx?t=SummaryReport&l=s |
Site collection web analytics reports | /_layouts/WebAnalytics/Report.aspx?t=SummaryReport&l=sc |
Site collection administration
Title | URL |
---|---|
Search settings | /_layouts/enhancedSearch.aspx |
Search scopes | /_layouts/viewscopes.aspx |
Search keywords | /_layouts/listkeywords.aspx |
Site level recycle bin | /_layouts/RecycleBin.aspx |
Site collection recycle bin | /_layouts/AdminRecycleBin.aspx |
Site collection features | /_layouts/ManageFeatures.aspx?Scope=Site |
Site directory settings | /_layouts/SiteDirectorySettings.aspx |
Site hierarchy | /_layouts/vsubwebs.aspx |
Site collection navigation | /_layouts/SiteNavigationSettings.aspx |
Site collection audit settings | /_layouts/AuditSettings.aspx |
Audit log reports | /_layouts/Reporting.aspx?Category=Auditing |
Portal site connection | /_layouts/portal.aspx |
Site collection policies | /_layouts/Policylist.aspx |
Storage Metrics | /_layouts/storman.aspx |
Record declaration settings | /_layouts/InPlaceRecordsSettings.aspx?Source=settings.aspx |
Content type publishing | /_Layouts/contenttypesyndicationhubs.aspx |
Site collection cache profiles | /_Layouts/RedirectPage.aspx?Target={SiteCollectionUrl}cache profiles |
Site collection object cache | /_Layouts/objectcachesettings.aspx |
Content type service application error log | /Lists/ContentTypeAppLog |
Site collection output cache | /_Layouts/sitecachesettings.aspx |
Variations | /_Layouts/VariationSettings.aspx |
Variation labels | /_Layouts/VariationLabels.aspx |
Translatable columns | /_Layouts/TranslatableSettings.aspx |
Variation logs | /_Layouts/VariationLogs.aspx |
Suggested Content Browser Locations | /PublishedLinks/ |
Document ID settings | /_Layouts/DocIdSettings.aspx |
SharePoint Designer Settings | /_layouts/SharePointDesignerSettings.aspx |
Visual Upgrade | /_layouts/suppux.aspx |
Help settings | /_layouts/HelpSettings.aspx |
Central administration
Title | URL |
---|---|
Manage web applications | /_admin/WebApplicationList.aspx |
Create site collections | /_admin/createsite.aspx |
Manage service applications | /_admin/ServiceApplications.aspx |
Manage content databases | /_admin/CNTDBADM.aspx |
Check job status | /_admin/Timer.aspx |
Manage farm administrators group | /_layouts/people.aspx?MembershipGroupId=3 |
Configure service accounts | /_admin/FarmCredentialManagement.aspx |
Configure send to connections | /_admin/OfficialFileAdmin.aspx |
Configure content deployment paths and jobs | /_admin/Deployment.aspx |
Manage form templates | _admin/ManageFormTemplates.aspx |
Manage servers in farm | /_admin/FarmServers.aspx |
Manage services on server | /_admin/Server.aspx |
Manage farm features | /_admin/ManageFarmFeatures.aspx |
Configure alternate access mappings | /_admin/AlternateUrlCollections.aspx |
Perform a backup | /_admin/Backup.aspx |
Restore from backup | /_admin/BackupHistory.aspx?restore=1&filter=1 |
Perform site collection backup | /_admin/SiteCollectionBackup.aspx |
Convert farm license type | /_admin/Conversion.aspx |
Check product and patch installation status | /_admin/PatchStatus.aspx |
Check upgrade status | /_admin/UpgradeStatus.aspx |
Common actions
Title | URL |
---|---|
Create new |
_layouts/spscreate.aspx _layouts/create.aspx |
View lists | _layouts/viewlsts.aspx |
Create site groups | _layouts/permsetup.aspx |
Quick launch | _layouts/quiklnch.aspx |
Workflow history list | /lists/Workflow History |
SharePoint server version + patch level | /_vti_pvt/Service.cnf (run at site collection level) |
Web part maintenance mode |
?Contents=1 (classic) ?maintenancemode=true (modern) |
Save as site template | _layouts/savetmpl.aspx |
Manage user alerts | _layouts/AlertsAdmin.aspx |
REST API examples
Operation | SharePoint REST API endpoint |
---|---|
Site | |
Get a SharePoint Site Collection | [domain]/_api/site |
Get a specific site or web | [domain]/_api/web |
Get a sites title | [domain]/_api/title |
List | |
Get all lists from a site | [domain]/_api/web/lists |
Get all items from a list | [domain]/_api/web/lists/getbytitle(listname)/items |
Get a lists title | [domain]/_api/web/lists/getbytitle(listname)?select=Title |
Get all columns within a list | [domain]/_api/web/lists/getbytitle(listname)/Fields |
Get list from GUID | [domain]/_api/Web/Lists(list GUID here) |
Get list item by ID | [domain]/_api/Web/Lists/GetByTitle/(listtitle)/GetItemById(2) |
Get selected fields for list items | [domain]/_api/Web/Lists/GetByTitle/(listtitle)/Items?select=ID,Title |
User | |
Get current user information | [domain]/_api/web/currentUser |
Get all site users | [domain]/_api/Web/siteusers |
Group | |
Get all groups from the site | [domain]/_api/Web/siteusers/sitegroups |
Get group by group ID | [domain]/_api/Web/sitegroups/GetById(GroupId) |
Get all users from group | [domain]/_api/Web/sitegroups(Id)/users |
Tuesday, September 12, 2023
Extract all URLs from a web page
Here is a quick JavaScript snippet to extract all URLs from a webpage, using Developer Tools. No Browser Extension is required!
Monday, September 4, 2023
Get icon from image file - CSS
CSS to get Carret symbol
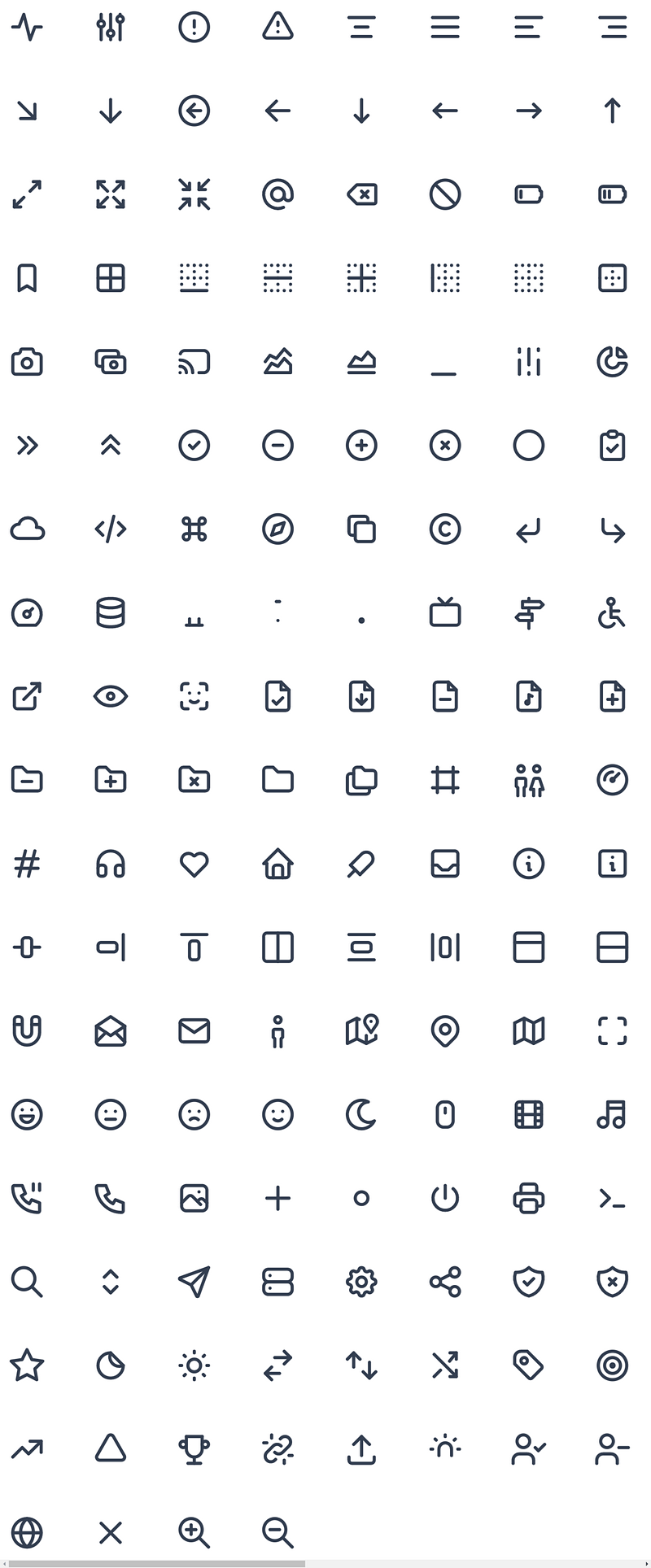
Friday, July 21, 2023
Clone columns of the table using jQuery
Following is the example where you can clone required columns and sequence from the source table to the new table
Monday, July 10, 2023
Read File Content from SharePoint List Items
Working function, where you can read file content available as an attachment in your SharePoint list item.
#sharepoint #jquery #sharepointsolutions
Tuesday, July 4, 2023
VS-Code Cheatsheet
BASICS
Ctrl+Shift+N
New window/instance
Ctrl+Shift+W
Close window/instance
Ctrl+Shift+P or F1
Show Command Palette
Ctrl+P
Quick Open, Go to File
Ctrl+,
User Settings
Ctrl+K Ctrl+S
Keyboard Shortcuts
Ctrl+C
Copy line (empty selection)
Ctrl+X
Cut line (empty selection)
Alt+ ↑ / ↓
Move line up/down
Shift+Alt + ↓ / ↑
Copy line up/down
Ctrl+Shift+K
Delete line
Ctrl+Shift+Enter
Insert line above
Ctrl+Home
Go to beginning of file
Ctrl+End
Go to end of file
Home / End
Go to beginning/end of line
Ctrl+↑ / ↓
Scroll line up/down
Ctrl+Shift+\
Jump to matching bracket
Ctrl+Enter
Insert line below Basic editing
Ctrl+] / [
Indent/outdent line
Ctrl+Shift+[
Fold (collapse) region
Ctrl+Shift+]
Unfold (uncollapse) region
Alt+PgUp / PgDn
Scroll page up/down Basic editing
Ctrl+/
Toggle line comment
Alt+Z
Toggle word wrap
Ctrl+K Ctrl+U
Remove line comment Navigation
Ctrl+T
Show all Symbols
Ctrl+P
Go to File...
Ctrl+K Ctrl+[
Fold (collapse) all subregions
Ctrl+K Ctrl+0
Fold (collapse) all regions
Ctrl+K Ctrl+J
Unfold (uncollapse) all regions
Ctrl+K Ctrl+]
Unfold (uncollapse) all subregions
Ctrl+K Ctrl+C
Add line comment
Ctrl+Shift+O
Go to Symbol
Ctrl+G
Go to Line Navigation
Ctrl+Shift+M
Show Problems panel
Shift+F8
Go to previous error or warning
Ctrl+Shift+Tab
Navigate editor group history
F8
Go to next error or warning
SEARCH AND REPLACE
Ctrl+F
Find
F3 / Shift+F3
Find next/previous
Alt+Enter
Select all occurences of Find match
Ctrl+H
Replace Search and replace
Ctrl+D
Add selection to next find match
Alt+C / R / W
Toggle case
Ctrl+K Ctrl+D
Move last selection to next find match
MULTI CURSOR SELECTION
Alt+Click
Insert cursor
Ctrl+U
Undo last cursor operation
Shift+Alt+I
Insert cursor at end of each line selected
Ctrl+Alt+ ↑ / ↓
Insert cursor above / below
Ctrl+L
Select current line
Ctrl+F2
Select all occurrences of current word
Shift+Alt+ →
Expand selection
Ctrl+Shift+L
Select all occurrences of current selection
Shift+Alt+ ←
Shrink selection
Ctrl+Shift+Alt + (arrow key)
Column (box) selection
Ctrl+Shift+Alt + PgUp/PgDn
Column (box) selection page up/down
Shift+Alt + (drag mouse)
Column (box) selection
LANGUAGE EDITING
F2
Rename
F12
Go to Definition
Ctrl+K F12
Open Definition to the side
Ctrl+.
Quick Fix
Alt+F12
Peek Definition
Ctrl+Space, Ctrl+I
Trigger suggestion
Shift+Alt+F
Format document
Ctrl+K Ctrl+F
Format selection
Ctrl+Shift+Space
Trigger parameter hints
Shift+F12
Show References
Ctrl+K Ctrl+X
Trim trailing whitespace
Ctrl+K M
Change file language
EDITOR
Ctrl+K Ctrl+ ←/→
Focus into previous/next editor group
Ctrl+K ← / →
Move active editor group
Ctrl+F4, Ctrl+W
Close editor
Ctrl+\
Split editor
Ctrl+ 1 / 2 / 3
Focus into 1st, 2nd or 3rd editor group
Ctrl+K F
Close folder
Ctrl+Shift+PgUp / PgDn
Move editor left/right
Ctrl+M
Toggle Tab moves
Shift+Alt+A
Toggle block comment
FILE MANAGEMENT
Ctrl+N
New File
Ctrl+S
Save
Ctrl+Shift+S
Save As
Ctrl+O
Open File
Ctrl+K S
Save All
Ctrl+K Ctrl+W
Close All
Ctrl+Shift+T
Reopen closed editor
Ctrl+F4
Close
Ctrl+K
Enter Keep preview mode editor open
Ctrl+Shift+Tab
Open previous
Ctrl+K P
Copy path of active file
Ctrl+Tab
Open next
Ctrl+K R
Reveal active file in Explorer
Ctrl+K O
Show active file in new window/instance
DISPLAY
F11
Toggle full screen
Ctrl+Shift+E
Show Explorer / Toggle focus
Ctrl+Shift+G
Show Source Control
Ctrl+Shift+D
Show Debug
Ctrl+Shift+F
Show Search Display
Ctrl+Shift+X
Show Extensions
Ctrl+Shift+J
Toggle Search details
Ctrl+Shift+U
Show Output panel
Ctrl+Shift+H
Replace in files
Ctrl+Shift+V
Open Markdown preview
Ctrl+ +/
Zoom in/out
Ctrl+B
Toggle Sidebar visibility
Shift+Alt+0
Toggle editor layout (horizontal/vertical)
Ctrl+K Z
Zen Mode (Esc, Esc to exit)
Ctrl+K V
Open Markdown preview to the side
DEBUGGING
F5
Start/Continue Debug
F9
Toggle breakpoint
F10
Step over
Shift+F5
Stop
F11 / Shift+F11
Step into/out
Ctrl+K Ctrl+I
Show hover Integrated terminal
Ctrl+`
Show integrated terminal
Ctrl+C
Copy selection
Ctrl+V
Paste into active terminal
Ctrl+Shift+`
Create new terminal Integrated terminal
Ctrl+↑ / ↓
Scroll up/down
Ctrl+Home /
End Scroll to top/bottom
Shift+PgUp / PgDn
Scroll page up/down
Free resources links on web development over the internet
Free resources on web development
ONLINE LEARNING PLATFORM- Free Code Camp https://www.freecodecamp.org/
- Udemy https://www.udemy.com/
- Treehouse https://teamtreehouse.com/
- Udacity https://www.udacity.com/
- Cousera https://www.coursera.org/
- Sololearn https://www.sololearn.com/
- Frontend Masters https://frontendmasters.com/
- W3Schools https://www.w3schools.com/
- Codeacademy https://www.codecademy.com/
- Traversy Media https://www.traversymedia.com/
- FreeCodeCamp https://youtube.com/c/Freecodecamp
- Traversy Media https://youtube.com/c/TraversyMedia
- Dev Ed https://youtube.com/c/DevEd
- Programming With Mosh https://youtube.com/c/programmingwithmosh
- Tyler Moore https://youtube.com/user/Conutant
- Web Bos https://youtube.com/c/WesBos
- Coding The Smart Way https://youtube.com/c/Codingthesmartway
- Code With Harry https://youtube.com/c/CodeWithHarry
- Clever Programmer https://youtube.com/c/CleverProgrammer
- Yahoo Baba https://youtube.com/c/YahooBaba
- Design Course https://youtube.com/c/DesignCourse
- Dev Docs https://devdocs.io/
- GeeksForGeeks https://devdocs.io/
- Tutorials Point https://www.tutorialspoint.com/
- W3Schools https://www.w3schools.com/
- Java Point https://www.javatpoint.com/
- Programming Hub https://programminghub.io/
- Grasshopper https://careerkarma.com/blog/grasshopper-app-review/
- Encode https://play.google.com/store/apps/details?id=com.upskew.encode&hl=en
- Mimo https://getmimo.com/
- Programming Hero https://www.programming-hero.com/
- Sololearn https://www.sololearn.com/
- Khan Academy https://www.khanacademy.org/computing/computer-programming
- W3Schools https://www.w3schools.com/
- CSS Tricks https://css-tricks.com/
- Smashing Magazine https://www.smashingmagazine.com/
- Site Point https://www.sitepoint.com/
- Dev Community https://dev.to/
- Evanto Tuts https://tutsplus.com/
- Medium https://medium.com/
- Over API https://overapi.com/
- Awesome Cheat Sheets https://lecoupa.github.io/awesome-cheatsheets/
- HTML5 Element Index http://html5doctor.com/element-index/
- HTML CheatSheet https://htmlcheatsheet.com/
- Can I Use https://caniuse.com/
- CSS Animation http://www.justinaguilar.com/animations/
- CSS Grid Cheat Sheet https://grid.malven.co/
- CSS Flexbox Cheat Sheet https://flexbox.malven.co/
- CSS Easing Functions https://easings.net/
- Web Development Learn By Doing HTML5 & CSS3 From Scratch https://www.u
- Master The Basics Of HTML5 & CSS3 Beginner Web Development https://www.udemy.com/c
- HTML And CSS Foundations https://www.udemy.com/course/html-and-css-foundations/
- Build Your First Website In 1 Week https://www.udemy.com/course/build-your-first-website-in-1-week/
- Introduction To CSS Beginners Guide To CSS Web Design https://www.udemy.com/course
- CSS Pocket Reference https://drive.google.com/file/d/1fqKiCvkESei14Oh3RoGCBBJkewZ3-
- CSS3 The Missing Manual https://drive.google.com/file/d/1deKgQjs9O0MoivQvDH3wyLz_nrU
- Head First HTML & CSS https://drive.google.com/file/d/1Ig31aq3gEd6qyOuLuvL1aeJXlE_A1B
- HTML & CSS Design & Build Websites - https://drive.google.
- HTML5 https://drive.google.com/file/d/1nxYanqOOsA3olZkfIL5BZsMoax_MMQ0R/vi ew?
- CSS3 https://drive.google.com/file/d/1clN8z5fH0cjR4NiBZTNXbS6dfw0GOjhr/vie w?usp=shari
- Codepen https://codepen.io/
- Free Frontend https://freefrontend.com/
- Code My UI https://codemyui.com/
- Play Code https://playcode.io/
- JS Bin https://jsbin.com/
- JS Fiddle https://jsfiddle.net/
- CodeSandBox https://codesandbox.io/
- Web Code Tools https://webcode.tools/
- CSS Flow https://www.cssflow.com/
- CodePly https://www.codeply.com/
- CodePad https://coderpad.io/
- CSS Deck https://cssdeck.com/
- Code Interview https://codeinterview.io/
- GitHub Pages https://github.com/
- Netlify https://www.netlify.com/
- AWS https://aws.amazon.com/
- Firebase https://firebase.google.com/
- Infinity Free https://infinityfree.net/
- WordPress https://wordpress.com/
- Wix https://www.wix.com/
- Weebly https://www.weebly.com/in
- Shopify https://www.shopify.in/
- TopCoder https://www.topcoder.com/challenges/?pageIndex=1
- Coderbyte https://www.coderbyte.com/
- HackerRank https://www.hackerrank.com/domains
- CodeChef https://www.codechef.com/
- Codewars https://www.codewars.com/
- LeetCode https://leetcode.com/
- Floxbox Defense http://www.flexboxdefense.com/
- Unflod https://unfold.com/
- Grid Garden https://cssgridgarden.com/
- CSS Diner https://flukeout.github.io/
- Code Combat https://codecombat.com/
- Coding Game https://www.codingame.com/
- Screeps https://screeps.com/
- Flexbox Froggy https://flexboxfroggy.com/
- Code Monkey https://www.codemonkey.com/
- Cyber Dojo https://www.cyber-dojo.org/creator/home
- CSS Grid Generator https://cssgrid-generator.netlify.app/
- CSS Flexbox Generator https://cssflex-generator.netlify.app/
- CSS Matic https://www.cssmatic.com/
- Patternify http://www.patternify.com/
- ColorZilla Gradients https://www.colorzilla.com/gradient-editor/
- Gradient Generator https://cssgradient.io/
- CSS Layout Generator https://csslayout.io/
- Animation Generator https://cssanimate.com/
- CSS3 Generator https://css3generator.com/
- Web Code Tools https://webcode.tools/generators/css
- Templated https://themewagon.com/author/templated/
- Zero Theme https://www.zerotheme.com/
- UI Deck https://uideck.com/
- Admin UI Themes https://athemes.com/collections/free-bootstrap-admin-templates/
- HTML5 Up https://html5up.net/
- Theme Wagon https://themewagon.com/
- ColorLib https://colorlib.com/
- W3Layouts https://w3layouts.com/
- Awesome CSS https://github.com/awesome-css-group/awesome-css
- Web Developer Roadmap https://github.com/kamranahmedse/developer-roadmap
- JavaScript Algorithms https://github.com/trekhleb/javascript-algorithms
- Awesome Learning Resources https://github.com/lauragift21/awesome-learning-resources
- Clean Code JavaScript https://github.com/ryanmcdermott/clean-code-javascript
- Frontend Checklist https://github.com/thedaviddias/Front-End-Checklist
- JavaScript Concepts https://github.com/leonardomso/33-js-concepts
- 30 Seconds Of Code https://github.com/30-seconds/30-seconds-of-code
- CSS Protips https://github.com/AllThingsSmitty/css-protips
- Color Hunt https://colorhunt.co/
- HTML Color Code https://htmlcolorcodes.com/
- Coolors https://coolors.co/
- UI Gradients https://uigradients.com/
- Gradient Genarator https://www.colorzilla.com/gradient-editor/
- Flat UI Colors https://flatuicolors.com/
- CSS Peeper https://chrome.google.com/webstore/detail/css- peeper/mbnbehi
- Colorpick Eyedropper https://chrome.google.com/webstore/detail/
- Window Resizer https://chrome.google.com/webstore/detail/window- res
- Lorem Ipsum Generator https://chrome.google.com/webstore/d
- CSS Scan https://chrome.google.com/webstore/detail/css- scan/gieabiemggnpn
- CSS Viewer https://chrome.google.com/webstore/detail/css-v
- HTML Validator https://chrome.google.com/webstore/detail/k
- Wappalyzer https://chrome.google.com/webstore/detail/wappalyzer/gppongmhj
- JSON Viewer https://chrome.google.com/webstore/detail/json- 19
- viewer/gbmdgpbipfallnflgajpaliibnhdgobh?hl=en US
- Font Awesome https://fontawesome.com/
- Icons8 https://icons8.com/
- Flaticon https://www.flaticon.com/
- Pixeden https://www.pixeden.com/
- Fontello https://fontello.com/
- Icon Finder https://www.iconfinder.com/
- Captain Icon https://mariodelvalle.github.io/CaptainIconWeb/
- Endless Icons https://endlessicons.com/
- Icon Icons - https://icon-icons.com/
- Round Icons https://roundicons.com/
- Freepik https://www.freepik.com/
- Undraw https://undraw.co/
- Drawkit https://www.drawkit.io/
- Humaaans https://www.humaaans.com/
- Handz https://www.handz.design/
- Open Doodles https://www.opendoodles.com/
- Illustration. co http://illustration.co/
- Vivid Js https://webkul.github.io/vivid/
- Many Pixels https://www.manypixels.co/
- JavaScript Weekly https://javascriptweekly.com/
- Node.js Weekly https://nodeweekly.com/
- FrontEnd Focus https://frontendfoc.us/
- WebTools Weekly https://webtoolsweekly.com/
- CSS Weekly https://css-weekly.com/
- JavaScript Kicks https://javascriptkicks.com/
- Behance https://www.behance.net/
- Awwwards http://www.awwwards.com/
- CSS Nectar http://cssnectar.com/
- SiteInspire https://www.siteinspire.com/
- Frontend Mentor https://www.frontendmentor.io/
- Animate. CSS http://daneden.github.io/animate.css/
- Bounce JS http://bouncejs.com/
- Anime JS http://anime-js.com/
- CSShake http://elrumordelaluz.github.io/csshake/#1
- Hover. CSS http://ianlunn.github.io/Hover/
- Hidden Tools https://hiddentools.dev/
- Iconscout https://iconscout.com/
- CSS Zen Garden http://www.csszengarden.com/
- A List Apart https://alistapart.com/
- Code Project https://www.codeproject.com/
- Scotch https://scotch.io/
- The Odin Project https://www.theodinproject.com/
- CSS Autor https://cssauthor.com/
- Gradient Magic https://www.gradientmagic.com/
- Trianglify https://trianglify.io/
- CSS Reference https://cssreference.io/
- HTML Reference https://htmlreference.io/
- Free CSS https://www.free-css.com/
- Fun JavaScript Projects https://fun-javascript-projects.com/
- Daily Dev https://daily.dev/
- Dev Docs https://devdocs.io/
- What Runs https://www.whatruns.com/
- 1LOC https://1loc.dev/
- Am i Responsive http://ami.responsivedesign.is/
- Shape Divider https://www.shapedivider.app/
- Fancy Border Radius https://9elements.github.io/fancy-border-radius/
- CSS Clip Path https://bennettfeely.com/clippy/
- 30 Seconds Of Code https://www.30secondsofcode.org/
- Undraw https://undraw.co/
- Humaaans https://www.humaaans.com/